This tutorial explain with the simple application which explains,
- How to create sq-lite database
- How to insert data into sq-lite database
- How to retrieve the data from sq-lite and display it in ListView
- Using CursorAdapter (To perform Edit, Delete from list)
- Using CursorAdapter (To perform Edit, Delete from list)
To create this simple application do the following:-
To create sq-lite database
Create the class with the name DBHelper with extends SQLiteOpenHelper and copy the following coding
Check it in previous tutorial Sq-Lite and ListView(I)
Create the class with the name DBUtil and copy the following coding
public int deltask(String pid) { return sqliteDb.delete(DBHelper.TODO_TABLE, DBHelper.ROW_ID_FIELD + "='" + pid + "'", null);
Then create the activities
First Screen
MainActivity.java
public class MainActivity extends AppCompatActivity implements View.OnClickListener,MyCustomAdapter.Staffinterface{ Button btn1; ListView listview; private MyCustomAdapter dataAdapter; DBUtil dbUtil; Context context; @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_main); context=this; btn1 = (Button) findViewById(R.id.btnid); listview = (ListView) findViewById(R.id.listid); btn1.setOnClickListener(this); dbUtil = new DBUtil(this); dbUtil.open(); setlist(); } private void setlist() { Cursor cur = dbUtil.getalltask(); if (cur.moveToFirst() && cur != null) { cur.moveToLast(); int c = cur.getCount(); dataAdapter = new MyCustomAdapter(context,cur,MainActivity.this); listview.setAdapter(dataAdapter); Log.e("COUNT9",""+c); } else{ Toast.makeText(this, "No To-Do entry", Toast.LENGTH_SHORT).show(); } } @Override public void onClick(View v) { switch(v.getId()){ case R.id.btnid: /** Start a new Activity MyCards.java */ Intent intent = new Intent(this, AddTodo.class); this.startActivity(intent); break ; } } public void staffdelete(final String pid) { Log.e("row id ",""+pid); //here call delete query then call setlist(); dbUtil.deltask(pid); setlist(); } }
activity_main.xml
<?xml version="1.0" encoding="utf-8"?>
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:paddingBottom="@dimen/activity_vertical_margin"
android:paddingLeft="@dimen/activity_horizontal_margin"
android:paddingRight="@dimen/activity_horizontal_margin"
android:paddingTop="@dimen/activity_vertical_margin"
tools:context="com.example.mrbrown.todo.MainActivity">
<LinearLayout
android:id="@+id/lineid"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:background="#6666ff"
>
<Button
android:id="@+id/btnid"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="ADD"
android:layout_marginLeft="270dp"
/>
</LinearLayout>
<ListView
android:id="@+id/listid"
android:layout_below="@+id/lineid"
android:layout_width="match_parent"
android:layout_height="wrap_content">
</ListView>
</RelativeLayout>
list_text_two.xml for ListView
<?xml version="1.0" encoding="utf-8"?>
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="fill_parent"
android:layout_height="wrap_content"
android:orientation="vertical"
android:padding="6dip" >
<LinearLayout
android:layout_width="match_parent"
android:layout_height="wrap_content">
<TextView
android:id="@+id/textView1"
android:layout_weight="2"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_alignParentLeft="true"
android:layout_alignParentTop="true"
android:visibility="gone"
android:textAppearance="?android:attr/textAppearanceMedium" />
<TextView
android:id="@+id/textView2"
android:layout_weight="20"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:textAppearance="?android:attr/textAppearanceMedium"
/>
<Button
android:layout_weight="2"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:text="X"/>
</LinearLayout>
</RelativeLayout>
MyCustomAdapter.java
public class MyCustomAdapter extends CursorAdapter { private LayoutInflater mInflater; private Staffinterface staffinter; public MyCustomAdapter(Context context, Cursor c, Staffinterface staffinter) { super(context, c); this.staffinter=staffinter; mInflater = LayoutInflater.from(context); } @Override public void bindView(final View arg0, Context arg1, Cursor arg2) { ViewHolder viewHolder = (ViewHolder) arg0.getTag(); if (viewHolder == null) { viewHolder = new ViewHolder(); viewHolder.tvName = (TextView) arg0.findViewById(R.id.textView1); viewHolder.tvFamily = (TextView) arg0.findViewById(R.id.textView2); viewHolder.btndelete=(Button) arg0.findViewById(R.id.btndelid); viewHolder.rid = arg2.getString(arg2 .getColumnIndexOrThrow(DBHelper.ROW_ID_FIELD)); viewHolder.task = arg2.getString(arg2 .getColumnIndexOrThrow(DBHelper.TASK_FIELD)); arg0.setTag(viewHolder); } final String rid = arg2.getString(arg2 .getColumnIndexOrThrow(DBHelper.ROW_ID_FIELD)); String tk = arg2.getString(arg2 .getColumnIndexOrThrow(DBHelper.TASK_FIELD)); viewHolder.tvName.setText(rid); viewHolder.tvFamily.setText(tk); viewHolder.btndelete.setOnClickListener(new View.OnClickListener() { @Override public void onClick(View v) { View vv = (View) v.getParent(); TextView tvName = (TextView) vv.findViewById(R.id.textView1); String vid = tvName.getText().toString(); Log.e("vid",""+vid); staffinter.staffdelete(vid); } }); } @Override public View getView(int position, View convertView, ViewGroup parent) { final View row = super.getView(position, convertView, parent); return row; } @Override public View newView(Context context, Cursor cursor, ViewGroup parent) { View v = mInflater.inflate(R.layout.list_text_two, null); return v; } static class ViewHolder { TextView tvName; TextView tvFamily; Button btndelete; String rid; String task; } public interface Staffinterface { public void staffdelete(String pid); } }
Second Screen
AddTodo.java
public class AddTodo extends Activity implements View.OnClickListener{
DBUtil dbUtil;
EditText edt;
Button btn2;
String stredt;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setTitle("Add Record");
setContentView(R.layout.add_todo);
edt = (EditText) findViewById(R.id.edtid);
btn2 = (Button) findViewById(R.id.btn2id);
btn2.setOnClickListener(this);
dbUtil = new DBUtil(this);
dbUtil.open();
}
@Override
public void onClick(View v) {
switch(v.getId()){
case R.id.btn2id: /** Start a new Activity MyCards.java */
stredt=edt.getText().toString();
if(stredt.length()>0){
dbUtil.addtask(stredt);
edt.setText("");
Intent intent = new Intent(this, MainActivity.class);
this.startActivity(intent);
}
break;
}
}
}
add_todo.xml
<?xml version="1.0" encoding="utf-8"?>
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:paddingBottom="@dimen/activity_vertical_margin"
android:paddingLeft="@dimen/activity_horizontal_margin"
android:paddingRight="@dimen/activity_horizontal_margin"
android:paddingTop="@dimen/activity_vertical_margin"
tools:context="com.example.mrbrown.todo.MainActivity">
<LinearLayout
android:id="@+id/lineid"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:background="#6666ff">
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="ADD"
android:layout_marginLeft="150dp"
android:textStyle="bold"
android:textSize="30dp"/>
</LinearLayout>
<EditText
android:id="@+id/edtid"
android:layout_below="@+id/lineid"
android:layout_marginTop="20dp"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:hint="Enter here..."/>
<Button
android:id="@+id/btn2id"
android:layout_below="@+id/edtid"
android:layout_width="100dp"
android:layout_height="wrap_content"
android:layout_marginLeft="125dp"
android:text="Submit"/>
</RelativeLayout>
Add WRITE_EXTERNAL_STORAGE to manifest
<uses-permission android:name="android.permission.WRITE_EXTERNAL_STORAGE"></uses-permission>
Manifest.xml
<?xml version="1.0" encoding="utf-8"?>
<manifest xmlns:android="http://schemas.android.com/apk/res/android"
package="com.example.mrbrown.todo">
<uses-permission android:name="android.permission.WRITE_EXTERNAL_STORAGE"></uses-permission>
<application
android:allowBackup="true"
android:icon="@mipmap/ic_launcher"
android:label="@string/app_name"
android:supportsRtl="true"
android:theme="@style/AppTheme">
<activity android:name=".MainActivity">
<intent-filter>
<action android:name="android.intent.action.MAIN" />
<category android:name="android.intent.category.LAUNCHER" />
</intent-filter>
</activity>
<activity android:name=".AddTodo">
<intent-filter>
<action android:name="android.intent.action.VIEW" />
<category android:name="android.intent.category.DEFAULT" />
</intent-filter>
</activity>
</application>
</manifest>
Output Screenshots
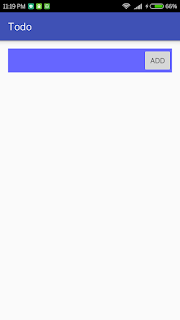
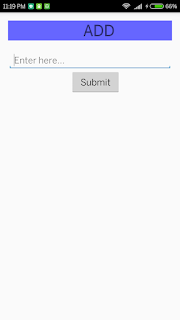
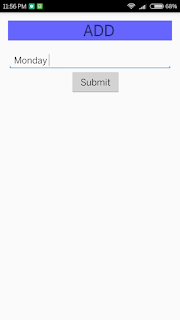
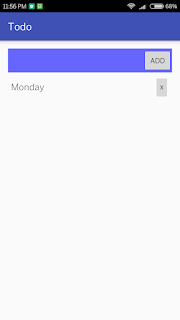
Source code for this application ToDoList.rar
________________________________________________________
Happy Coding...
Comments
Post a Comment