This tutorial explains how to generate bar-code or QR-code for the selling product
By
this application you can generate QR, Code 39, Code 128 and EAN13
How this codes get generated ?
Using
the library itextpdf-5.5.6.jar
I
have created a simple app to demonstrate this tutorial. Below is the
screenshot of the app which contains 5 buttons, 2 edit box and 3
radio buttons.
Enter in Data field and Description field and select the radio button(where the description want to display) and touch your required code button.
Each type of bar code has their validation
You will get the bar code output in the form of PDF as given,
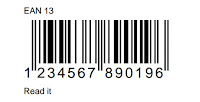
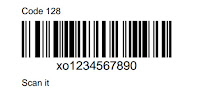
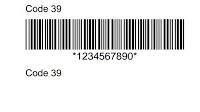
You can test the above codes by reading using the app Barcode Reader available in Google Play
Now
follow the coding to develop this simple application
1.
activity_main.xml
<LinearLayout
xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical"
android:layout_marginLeft="15dp"
android:layout_marginRight="15dp"
android:background="@color/greenish_brown">
<LinearLayout
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_gravity="center"
android:orientation="horizontal"
android:padding="5dp"
>
<TextView
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_weight="1"
android:text="Data"
/>
<EditText
android:id="@+id/data"
style="@style/Editbox"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_weight="1"
/>
</LinearLayout>
<LinearLayout
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_gravity="center"
android:orientation="horizontal"
android:padding="5dp"
>
<TextView
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_weight="1"
android:text="Description"
/>
<EditText
android:id="@+id/description"
style="@style/Editbox"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_weight="1"
/>
</LinearLayout>
<LinearLayout
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_gravity="center"
android:orientation="horizontal"
android:padding="5dp"
>
<TextView
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_weight="1"
android:text="Position
of Description" />
<RadioGroup
android:id="@+id/rbgroup"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_weight="1"
>
<RadioButton
android:id="@+id/rbbelow"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:checked="true"
android:text="Below"
/>
<RadioButton
android:id="@+id/rbabove"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Above"
/>
<RadioButton
android:id="@+id/rbnone"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="None"
/>
</RadioGroup>
</LinearLayout>
<Button
android:id="@+id/qrcode"
style="@style/BtnStyle4"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text="QRCode"
/>
<Button
android:id="@+id/code39"
style="@style/BtnStyle4"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_marginTop="5dp"
android:text="Code
39" />
<Button
android:id="@+id/code128"
style="@style/BtnStyle4"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_marginTop="5dp"
android:text="Code
128" />
<Button
android:id="@+id/ean13"
style="@style/BtnStyle4"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_marginTop="5dp"
android:text="EAN13"
/>
<Button
android:id="@+id/openpdf"
style="@style/BtnStyle5"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_marginTop="5dp"
android:text="Open
PDF" />
</LinearLayout>
2.
MainActivity.java
Use
the following coding in your MainActivity class
public
class MainActivity extends Activity {
private
Button btnqrcode;
private
Button btncode39;
private
Button btncode128;
private
Button btnean13;
private
Button btnopen;
private
EditText txtdata;
private
EditText txtdes;
private
RadioGroup rgroup;
private
RadioButton below;
private
RadioButton above;
private
RadioButton none;
private
String data = "";
private
String desc = "";
private
String pos = "Below";
private
PdfContentByte cb;
private
File file;
@Override
protected
void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
btnqrcode
= (Button) findViewById(R.id.qrcode);
btncode39
= (Button) findViewById(R.id.code39);
btncode128
= (Button) findViewById(R.id.code128);
btnean13
= (Button) findViewById(R.id.ean13);
btnopen
= (Button) findViewById(R.id.openpdf);
txtdata
= (EditText) findViewById(R.id.data);
txtdes
= (EditText) findViewById(R.id.description);
rgroup
= (RadioGroup) findViewById(R.id.rbgroup);
below
= (RadioButton) findViewById(R.id.rbbelow);
above
= (RadioButton) findViewById(R.id.rbabove);
none
= (RadioButton) findViewById(R.id.rbnone);
String
path = "";
Give
the path for PDF
rgroup.setOnCheckedChangeListener(new
OnCheckedChangeListener() {
@Override
public
void onCheckedChanged(RadioGroup arg0, int arg1) {
if
(below.isChecked() == true) {
pos
= "Below";
}
else if (above.isChecked() == true) {
pos
= "Above";
}
else if (none.isChecked() == true) {
txtdes.setText("");
pos
= "None";
}
}
});
btnqrcode.setOnClickListener(new
OnClickListener() {
@Override
public
void onClick(View arg0) {
try
{
data
= txtdata.getText().toString();
desc
= txtdes.getText().toString();
boolean
flag = true;
if
(pos.equals("None")) {
if
(data.length() > 0) {
flag
= true;
}
else {
flag
= false;
}
}
else {
if
(data.length() > 0 && desc.length() > 0) {
flag
= true;
}
else {
flag
= false;
}
}
if
(flag == true) {
Document
doc = new Document(new Rectangle(400, 500));
PdfWriter
writer = PdfWriter.getInstance(doc,
new
FileOutputStream(file));
doc.open();
cb
= writer.getDirectContent();
doc.add(new
Paragraph("QRCode"));
BarcodeQRCode
qrcode = new BarcodeQRCode(data, 1, 1,
null);
Image
img4 = qrcode.getImage();
img4.scaleToFit(100,
100);
if
(pos.equals("Above") && !desc.equals(""))
{
doc.add(new
Paragraph(desc));
doc.add(img4);
}
else if (pos.equals("Below") && !desc.equals(""))
{
doc.add(img4);
doc.add(new
Paragraph(desc));
}
else if (pos.equals("None")) {
doc.add(img4);
}
doc.close();
Toast.makeText(getApplicationContext(),
"QRCode
Created...", Toast.LENGTH_LONG).show();
txtdata.setText("");
txtdes.setText("");
}
else {
Toast.makeText(getApplicationContext(),
"Please
enter all data...", Toast.LENGTH_LONG)
.show();
}
}
catch (Exception e) {
}
}
});
btncode39.setOnClickListener(new
OnClickListener() {
@Override
public
void onClick(View v) {
try
{
data
= txtdata.getText().toString();
desc
= txtdes.getText().toString();
boolean
flag = true;
if
(pos.equals("None")) {
if
(data.length() > 0) {
flag
= true;
}
else {
flag
= false;
}
}
else {
if
(data.length() > 0 && desc.length() > 0) {
flag
= true;
}
else {
flag
= false;
}
}
if
(flag == true) {
Document
doc = new Document(new Rectangle(400, 500));
PdfWriter
writer = PdfWriter.getInstance(doc,
new
FileOutputStream(file));
doc.open();
cb
= writer.getDirectContent();
doc.add(new
Paragraph("Code 39"));
Barcode39
code39 = new Barcode39();
code39.setCode(data);
Image
img3 = code39.createImageWithBarcode(cb, null,
null);
img3.scaleToFit(200,
200);
if
(pos.equals("Above") && !desc.equals(""))
{
doc.add(new
Paragraph(desc));
doc.add(img3);
}
else if (pos.equals("Below") && !desc.equals(""))
{
doc.add(img3);
doc.add(new
Paragraph(desc));
}
else if (pos.equals("None")) {
doc.add(img3);
}
doc.close();
Toast.makeText(getApplicationContext(),
"Code
39 Created...", Toast.LENGTH_LONG).show();
txtdata.setText("");
txtdes.setText("");
}
else {
Toast.makeText(getApplicationContext(),
"Please
enter all data...", Toast.LENGTH_LONG)
.show();
}
}
catch (Exception e) {
}
}
});
btncode128.setOnClickListener(new
OnClickListener() {
@Override
public
void onClick(View v) {
try
{
data
= txtdata.getText().toString();
desc
= txtdes.getText().toString();
boolean
flag = true;
if
(pos.equals("None")) {
if
(data.length() > 0) {
flag
= true;
}
else {
flag
= false;
}
}
else {
if
(data.length() > 0 && desc.length() > 0) {
flag
= true;
}
else {
flag
= false;
}
}
if
(flag == true) {
Document
doc = new Document(new Rectangle(400, 500));
PdfWriter
writer = PdfWriter.getInstance(doc,
new
FileOutputStream(file));
doc.open();
cb
= writer.getDirectContent();
doc.add(new
Paragraph("Code 128"));
Barcode128
code128 = new Barcode128();
code128.setCode(data);
Image
img2 = code128.createImageWithBarcode(cb, null,
null);
img2.scaleToFit(200,
200);
if
(pos.equals("Above")) {
doc.add(new
Paragraph(desc));
doc.add(img2);
}
else if (pos.equals("Below")) {
doc.add(img2);
doc.add(new
Paragraph(desc));
}
else {
doc.add(img2);
}
doc.close();
Toast.makeText(getApplicationContext(),
"Code
128 Created...", Toast.LENGTH_LONG)
.show();
txtdata.setText("");
txtdes.setText("");
}
else {
Toast.makeText(getApplicationContext(),
"Please
enter all data...", Toast.LENGTH_LONG)
.show();
}
}
catch (Exception e) {
}
}
});
btnean13.setOnClickListener(new
OnClickListener() {
@Override
public
void onClick(View v) {
try
{
data
= txtdata.getText().toString();
desc
= txtdes.getText().toString();
boolean
flag = true;
String
error="";
if
(txtdata.length() > 0) {
if
(txtdata.length() >= 13) {
if
((data.matches("[0-9]+$")))
{
error
= null;
}
else {
error
= "Enter a valid Data e.g. : 1255684521453";
}
}
else {
error
= "Maximum of 13 characters are allowed";
}
}
else {
error
= "Enter a Data";
}
txtdata.setError(error);
if
(pos.equals("None")) {
if
(data.length() > 0) {
flag
= true;
}
else {
flag
= false;
}
}
else {
if
(data.length() > 0 && desc.length() > 0) {
flag
= true;
}
else {
flag
= false;
}
}
if
(flag == true) {
Document
doc = new Document(new Rectangle(400, 500));
PdfWriter
writer = PdfWriter.getInstance(doc,
new
FileOutputStream(file));
doc.open();
cb
= writer.getDirectContent();
doc.add(new
Paragraph("EAN 13"));
BarcodeEAN
codeEAN = new BarcodeEAN();
codeEAN.setCode(data);
Image
img1 = codeEAN.createImageWithBarcode(cb, null,
null);
img1.scaleToFit(200,
200);
if
(pos.equals("Above")) {
doc.add(new
Paragraph(desc));
doc.add(img1);
}
else if (pos.equals("Below")) {
doc.add(img1);
doc.add(new
Paragraph(desc));
}
else {
doc.add(img1);
}
doc.close();
Toast.makeText(getApplicationContext(),
"EAN
13 Created...", Toast.LENGTH_LONG).show();
txtdata.setText("");
txtdes.setText("");
}
else {
Toast.makeText(getApplicationContext(),
"Please
enter all data...", Toast.LENGTH_LONG)
.show();
}
}
catch (Exception e) {
}
}
});
coding
on button for open PDF
}
}
______________________________________________________
APK for this application AllBarcode-Generator
For any other query or for source code feel free to mail - arnoldbrown009@gmail.com
Happy Coding...
Comments
Post a Comment