In this tutorial i will be demonstrating how to build simple android ListView. This article is about creating listview and launching new activity on selecting single list item.
Now follow the coding
1. Create the project
2. Extend your MainActivity class from ListActivity
3. Now we need a string resources file to store all list item labels. So create an XML file under values folder and name it as list_data.xml and paste the following code.
( Right Click on res/values ⇒ New ⇒ Android XML File)list_data.xml
<?xml version="1.0" encoding="utf-8"?>
<resources>
<string-array name="android_versions">
<item>Cupcake</item>
<item>Donut</item>
<item>Eclair</item>
<item>Froyo</item>
<item>Gingerbread</item>
<item>Honeycomb</item>
<item>Ice Cream Sandwich</item>
<item>Jelly Bean</item>
<item>KitKat</item>
<item>Lollipop</item>
</string-array>
</resources>
<resources>
<string-array name="android_versions">
<item>Cupcake</item>
<item>Donut</item>
<item>Eclair</item>
<item>Froyo</item>
<item>Gingerbread</item>
<item>Honeycomb</item>
<item>Ice Cream Sandwich</item>
<item>Jelly Bean</item>
<item>KitKat</item>
<item>Lollipop</item>
</string-array>
</resources>
4. In ListView each list item will be an xml layout, so we can customize each list item. Create an XML file under res/layout folder and name it as list_item.xml and type the following code. This xml layout will be single list item row.
( Right Click on res/layout ⇒ New ⇒ Android XML File)
<?xml version="1.0" encoding="utf-8"?>
<TextView xmlns:android="http://schemas.android.com/apk/res/android"
android:id="@+id/label"
android:layout_width="fill_parent"
android:layout_height="fill_parent"
android:padding="10dip"
android:textSize="16dip"
android:textStyle="bold" >
</TextView>
5. Now open your main activity java file (AndroidListViewActivity.java) and type the following code. In the following code i am importing all xml resources data and storing them in an Array. On the next step i am binding array to ListAdapter.
public class AndroidListViewActivity extends ListActivity {
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
String[] android_versions = getResources().getStringArray(
R.array.android_versions);
this.setListAdapter(new ArrayAdapter<String>(this, R.layout.list_item,
R.id.label, android_versions));
}
}
6. Now run your project you can see listview with list of array items. But on clicking single list item you can see no action. So we need to start new activity on selecting single list item.
7. Now create new activity class under src folder. Right Click on src/package folder ⇒ New ⇒ Class and name it as SingleListItem . (SingleListItem.java)
8. Open your AndroidListViewActivity.java and modify the code to following. In the following code i am getting the selected list item string(android name) and sending it to new Activity.
AndroidListViewActivity.java
public class AndroidListViewActivity extends ListActivity {
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
String[] android_versions = getResources().getStringArray(
R.array.android_versions);
this.setListAdapter(new ArrayAdapter<String>(this, R.layout.list_item,
R.id.label, android_versions));
ListView lv = getListView();
lv.setOnItemClickListener(new OnItemClickListener() {
public void onItemClick(AdapterView<?> parent, View view,
int position, long id) {
// selected item
String product = ((TextView) view).getText().toString();
// Launching new Activity on selecting single List Item
Intent i = new Intent(getApplicationContext(),
SingleListItem.class);
// sending data to new activity
i.putExtra("product", product);
startActivity(i);
}
});
}
}
Now in new activity we need to display the received from listview activity.
9. Create a new xml file under res/layout and name it as single_list_item_view.xml and type the following code. This XML file will be layout for SingleListItem.java
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:background="#000000"
android:orientation="vertical" >
<TextView
android:layout_width="fill_parent"
android:layout_height="wrap_content"
android:background="#00ff00"
android:padding="5dip"
android:text="Android Version"
android:textSize="25dip"
android:textStyle="bold" />
<TextView
android:id="@+id/product_label"
android:layout_width="fill_parent"
android:layout_height="wrap_content"
android:padding="10dip"
android:textColor="#ffffff"
android:textSize="25dip"
android:textStyle="bold" />
<ImageView
android:id="@+id/imgid"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_gravity="center"
android:padding="10dip" />
</LinearLayout>
10. Now open your second activity file i.e. SingleListItem.java and paste the following code.
public class SingleListItem extends Activity {
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
this.setContentView(R.layout.single_list_item_view);
//set name
TextView txtProduct = (TextView) findViewById(R.id.product_label);
//set image
ImageView imgand = (ImageView) findViewById(R.id.imgid);
Intent i = getIntent();
// getting attached intent data
String product = i.getStringExtra("product");
// displaying selected product name
if (product.contentEquals("Cupcake")) {
txtProduct.setText(product + " (version - " + 1.5 + ")");
imgand.setImageDrawable(getResources().getDrawable(
R.drawable.cupcake));
} else if (product.contentEquals("Donut")) {
txtProduct.setText(product + " (version - " + 1.6 + ")");
imgand.setImageDrawable(getResources()
.getDrawable(R.drawable.donut));
} else if (product.contentEquals("Eclair")) {
txtProduct.setText(product + " (version - " + "2.0, 2.1" + ")");
imgand.setImageDrawable(getResources().getDrawable(
R.drawable.eclair));
} else if (product.contentEquals("Froyo")) {
txtProduct.setText(product + " (version - " + 2.2 + ")");
imgand.setImageDrawable(getResources()
.getDrawable(R.drawable.froyo));
} else if (product.contentEquals("Gingerbread")) {
txtProduct.setText(product + " (version - " + 2.3 + ")");
imgand.setImageDrawable(getResources().getDrawable(
R.drawable.gingerbread));
} else if (product.contentEquals("Honeycomb")) {
txtProduct
.setText(product + " (version - " + "3.0, 3.1, 3.2" + ")");
imgand.setImageDrawable(getResources().getDrawable(
R.drawable.honeycomb));
} else if (product.contentEquals("Ice Cream Sandwich")) {
txtProduct.setText(product + " (version - " + 4.0 + ")");
imgand.setImageDrawable(getResources().getDrawable(
R.drawable.icecreamsandwich));
} else if (product.contentEquals("Jelly Bean")) {
txtProduct
.setText(product + " (version - " + "4.1, 4.2, 4.3" + ")");
imgand.setImageDrawable(getResources().getDrawable(
R.drawable.jellybean));
} else if (product.contentEquals("KitKat")) {
txtProduct.setText(product + " (version - " + 4.4 + ")");
imgand.setImageDrawable(getResources().getDrawable(
R.drawable.kitkat));
} else {
txtProduct.setText(product + " (version - " + "5.0, 5.0.1, 5.0.2"
+ ")");
imgand.setImageDrawable(getResources().getDrawable(
R.drawable.lollipop));
}
}
}
11. The final step is to add an entry of new activity name in AndroidManifest.xml file. Open you AndroidManifest.xml file and modify the code as below
<?xml version="1.0" encoding="utf-8"?>
<manifest xmlns:android="http://schemas.android.com/apk/res/android"
package="com.example.listviewtutorial"
android:versionCode="1"
android:versionName="1.0" >
<uses-sdk
android:minSdkVersion="8"
android:targetSdkVersion="19" />
<application
android:allowBackup="true"
android:icon="@drawable/app_icon"
android:label="@string/app_name"
android:theme="@style/AppTheme" >
<activity
android:name=".AndroidListViewActivity"
android:label="@string/app_name" >
<intent-filter>
<action android:name="android.intent.action.MAIN" />
<category android:name="android.intent.category.LAUNCHER" />
</intent-filter>
</activity>
<activity android:name=".SingleListItem"
android:label="Single Item Selected"></activity>
</application>
</manifest>
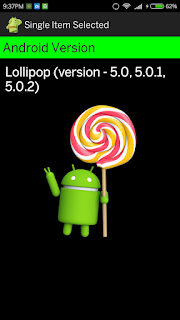
_________________________________________________________________
Source code for this application ListViewTutorial.zip
Happy Coding...
Comments
Post a Comment